回调函数在模型训练和模型学习结果分析中有非常重要的作用,如动态学习率调整,早停,tensorboard可视化分析模型训练过程。
Tensorboard可视化
1 | from keras.callbacks import TensorBoard |
嵌入层效果图:
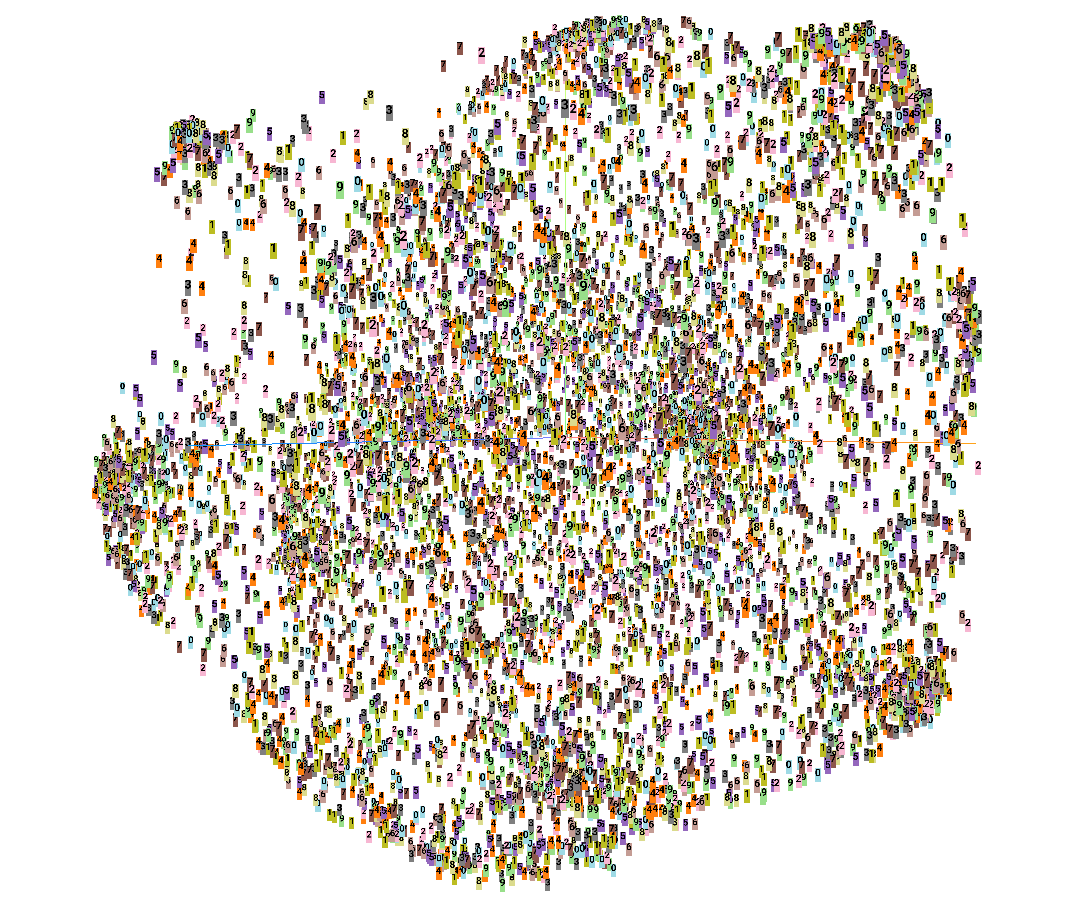
编写自己的可视化回调函数示例—PR曲线
代码参考
1 | from keras.callbacks import TensorBoard |
效果图展示:
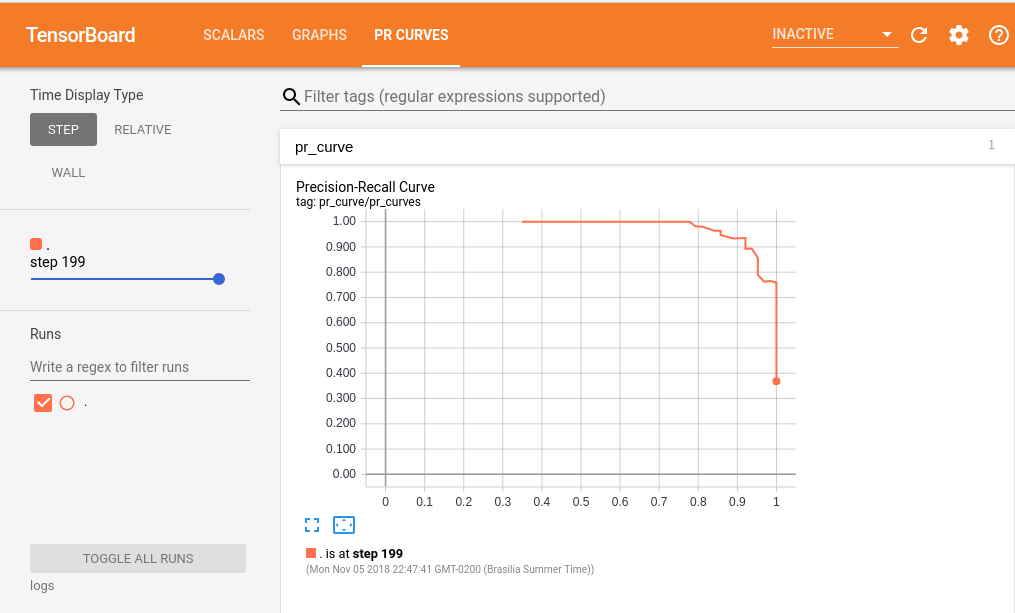
ModelCheckpoint
1 | keras.callbacks.ModelCheckpoint(filepath, monitor='val_loss', verbose=0, save_best_only=False, save_weights_only=False, mode='auto', period=1) |
EarlyStopping
1 | keras.callbacks.EarlyStopping(monitor='val_loss', min_delta=0, patience=0, verbose=0, mode='auto') |
LearningRateScheduler
1 | keras.callbacks.LearningRateScheduler(schedule, verbose=0) |
Scheduler示例
1 | callbacks = [] |
创建自定义回调函数
简单的创建使用LambdaCallback
1 | keras.callbacks.LambdaCallback(on_epoch_begin=None, on_epoch_end=None, on_batch_begin=None, on_batch_end=None, on_train_begin=None, on_train_end=None) |
1 | #示例 在每一个批开始时,打印出批数。 |
通过继承回调函数基类定义回调函数
1 | #在训练时,保存一个列表的批量损失值: |
Reference
所有参考均以贴上链接